Native
Android library for integrating WeFitter and Health Connect into your app
Installation
Add wefitter-health-connect-0.0.3.aar
to libs
folder which should normally be in app/libs
.
Add the following dependencies to your app/build.gradle
:
Hide code example
dependencies {
implementation fileTree(dir: 'libs', include: ['*.aar'])
implementation 'androidx.health.connect:connect-client:1.1.0-alpha07'
implementation 'androidx.lifecycle:lifecycle-runtime-ktx:2.7.0'
implementation 'androidx.security:security-crypto:1.0.0'
implementation 'androidx.work:work-runtime-ktx:2.9.0'
implementation 'com.auth0.android:jwtdecode:2.0.2'
implementation 'com.google.code.gson:gson:2.10.1'
implementation 'com.squareup.okhttp3:okhttp:4.11.0'
}
Usage
Add the following to your MainActivity.kt
:
Hide code example
import com.wefitter.healthconnect.WeFitterHealthConnect
import com.wefitter.healthconnect.WeFitterHealthConnectError
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// initialize WeFitter for Health Connect
val client = WeFitterHealthConnect(this)
// enter the token from the `bearer` field you receive in the response when a WeFitter profile was created via the [WeFitter API](https://www.wefitter.com/en-us/developers/documentation/#operation/profile_create)
val token = "BEARER_TOKEN"
setContent {
var checkedState by remember { mutableStateOf(false) }
var errorMessage by remember { mutableStateOf("") }
// create statusListener to receive status changes and errors
val statusListener = object : WeFitterHealthConnect.StatusListener {
override fun onConfigured(configured: Boolean) {
Log.i(WeFitterHealthConnect.TAG, "configured: $configured")
}
override fun onConnected(connected: Boolean) {
Log.i(WeFitterHealthConnect.TAG, "connected: $connected")
checkedState = connected
}
override fun onError(error: WeFitterHealthConnectError) {
Log.e(WeFitterHealthConnect.TAG, "error: ${error.message}")
errorMessage = error.message
}
}
// `configure` should be called every time your app opens
client.configure(
token = token,
statusListener = statusListener
)
WeFitterForHealthConnectTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background,
) {
Column(
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Switch(checked = checkedState, onCheckedChange = {
if (it) {
client.connect()
} else {
client.disconnect()
}
})
Text(
text = errorMessage, color = Color.Red
)
}
}
}
}
}
}
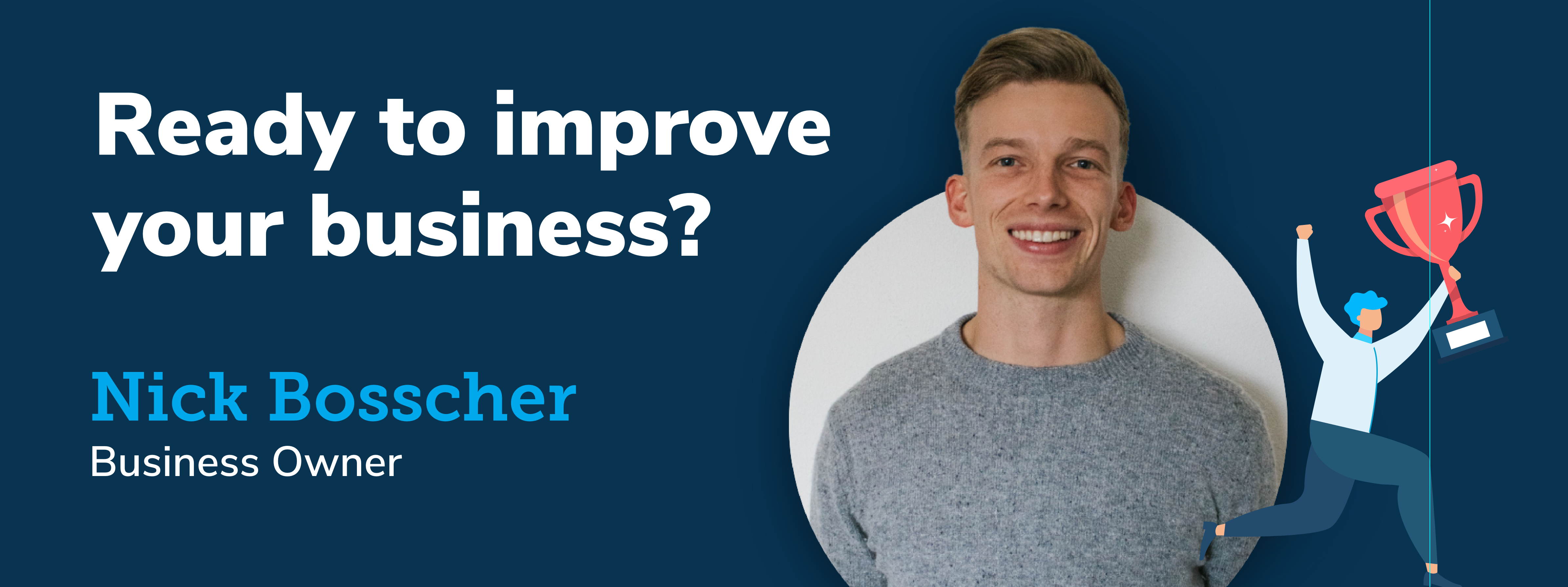