React Native
React Native library for integrating WeFitter and Health Connect into your app
- This library is written with Native Modules for React Native CLI. Expo Go is unsupported
Installation
Add wefitter-health-connect-0.1.2.aar
to libs
folder which should normally be in app/libs
. These files can be found in node_modules/react-native-wefitter-health-connect/android/libs
in your protect or here. Make sure you select the same version tag you are using in your app before downloading to prevent incompatibility issues.
Usage
Add the following code:
Breaking changes:
1. You can specify your app's permissions as shown in the example to configure the WeFitter SDK. By doing this, the user only sees the permissions for which your app has been approved. If you do not specify these permissions, ALL permissions will be presented to the user, thus also permissions for which you app has not been approved.
2. You have to request the android.Manifest.permission.ACTIVITY_RECOGNITION permission before you connect the WeFitter SDK, as without this permission the WeFitter SDK will never connect.
See the example app for the full source.
import WeFitterHealthConnect, {
ConfiguredEvent,
ConnectedEvent,
ErrorEvent,
} from 'react-native-wefitter-health-connect';
// ...
export default function App() {
const [connected, setConnected] = useState<boolean>(false);
const [configured, setConfigured] = useState<boolean>(false);
const [error, setError] = useState<ErrorEvent>();
const prefix = 'android.permission.health';
const myAppPermissions: string[] = [
`${prefix}.READ_DISTANCE,${prefix}.READ_STEPS`,
`${prefix}.READ_TOTAL_CALORIES_BURNED`,
`${prefix}.READ_HEART_RATE`,
`${prefix}.READ_POWER`,
`${prefix}.READ_EXERCISE`,
//"$prefix.READ_BLOOD_GLUCOSE",
//"$prefix.READ_BLOOD_PRESSURE",
//"$prefix.READ_BODY_FAT",
//"$prefix.READ_BODY_TEMPERATURE",
`${prefix}.READ_HEIGHT`,
//"$prefix.READ_OXYGEN_SATURATION",'
`${prefix}.READ_WEIGHT`,
`${prefix}.READ_SPEED`,
`${prefix}.READ_SLEEP`,
];
const myAppPermissionsString = myAppPermissions.join(',');
// create config
const config = {
token: 'YOUR_BEARER_TOKEN', // required, WeFitter API profile bearer token
apiUrl: 'YOUR_API_URL', // optional, only use if you want to use your backend as a proxy and forward all API calls to the WeFitter API. Default: `https://api.wefitter.com/api/`
startDate: 'CUSTOM_START_DATE', // optional with format `yyyy-MM-dd`, by default data of the past 20 days will be uploaded
notificationTitle: 'CUSTOM_TITLE', // optional
notificationText: 'CUSTOM_TEXT', // optional
notificationIcon: 'CUSTOM_ICON', // optional, e.g. `ic_notification` placed in either drawable, mipmap or raw
notificationChannelId: 'CUSTOM_CHANNEL_ID', // optional
notificationChannelName: 'CUSTOM_CHANNEL_NAME', // optional
appPermissions: myAppPermissionsString,
};
useEffect(() => {
console.log(`WeFitterHealthConnect useEffect`);
if (Platform.OS === 'android') {
// create native event emitter and event listeners to handle status updates
const emitter = new NativeEventEmitter();
const configuredListener = emitter.addListener(
'onConfiguredWeFitterHealthConnect',
(event: ConfiguredEvent) => {
console.log(`WeFitterHealthConnect configured: ${event.configured}`);
setConfigured(event.configured);
}
);
const connectedListener = emitter.addListener(
'onConnectedWeFitterHealthConnect',
(event: ConnectedEvent) => {
console.log(`WeFitterHealthConnect connected: ${event.connected}`);
setConnected(event.connected);
}
);
const errorListener = emitter.addListener(
'onErrorWeFitterHealthConnect',
(event: ErrorEvent) => {
console.log(`WeFitterHealthConnect error: ${event.error}`);
setError(event);
}
);
console.log(`WeFitterHealthConnect configure`);
// configure WeFitterHealthConnect
WeFitterHealthConnect.configure(config);
return () => {
configuredListener.remove();
connectedListener.remove();
errorListener.remove();
};
}
return;
}, []);
const onPressConnectOrDisconnect = () => {
if (Platform.OS === 'android') {
WeFitterHealthConnect.isSupported((supported) => {
if (supported) {
connected
? WeFitterHealthConnect.disconnect()
: WeFitterHealthConnect.connect();
} else {
Alert.alert(
'Not supported',
'WeFitterHealthConnect is not supported on this device'
);
}
});
} else {
Alert.alert(
'Not supported',
'WeFitterHealthConnect is not supported on iOS'
);
}
};
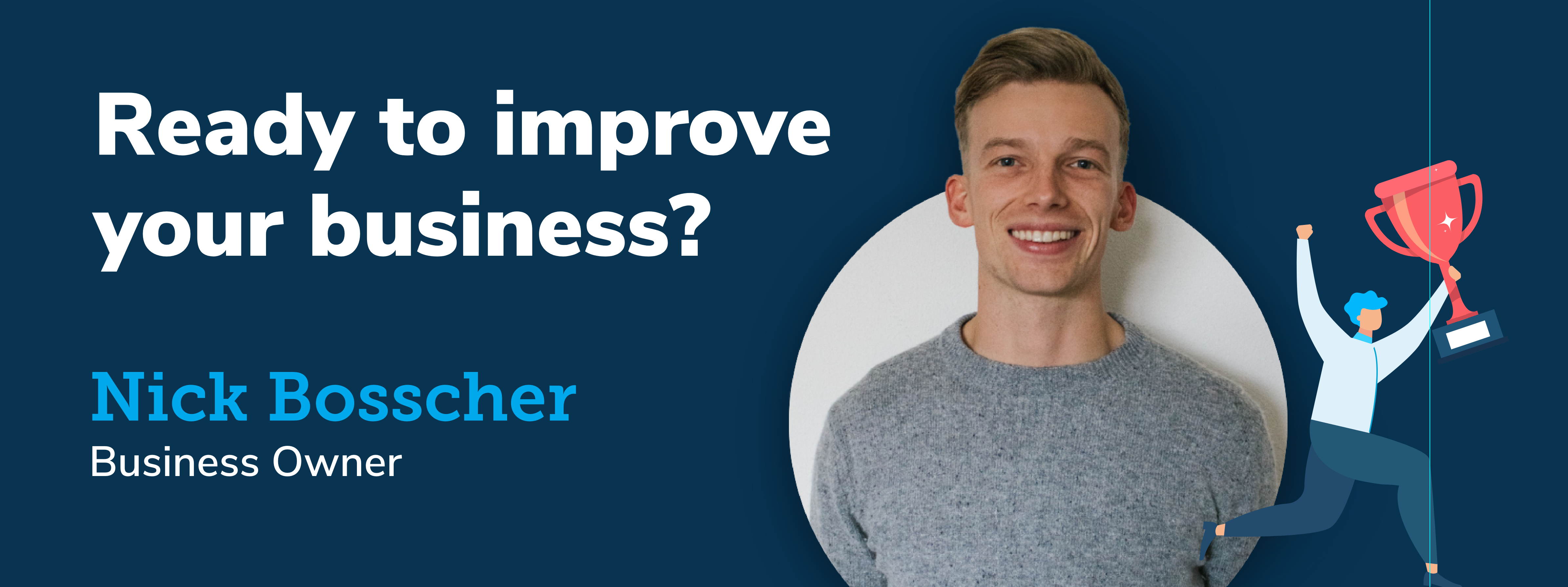